This post was viewed [wpstatistics stat=pagevisits time=total id=528] times.
Following a list of my preferred and maybe (?) best Visual Studio settings for modern C++ programming based on my experience and evaluations. Each mentioned setting will be explained with some short sentences.
I will cover “Release” Builds only – and I use Visual Studio 2022, but the same settings should be valid for Visual Studio 2019 as well. Settings which are unchanged are skipped (except they are very important to mention anyway).
All the settings are done via the “Property Page” dialog of the project.
We start with the page:
“Configuration Properties” ➜ “Advanced”
★ Set “Character Set” to “Use Unicode character Set”
This is Windows specific. But if we ever get in touch with the WIN32 API, we want to use the wide character variant as default and never ever deal with the ANSI variant and Code Pages at all!
Next page:
“Configuration Properties” ➜ “C/C++” ➜ “General”
★ Set “SDL checks” to “Yes”
This enables some security checks which leads to extra warnings or even compile errors if dangerous things are used. For example, the following code will not compile and must be modified to make the desired usage explicit.
unsigned int x = 1;
unsigned int foo = -x; // compile error b/c x is unsigned and foo is still unsigned but use unary minus.
// must make it explicit:
unsigned int foo = static_cast<unsigned int>( - static_cast<signed int>(x) ); // now compiles.
★ Set “Multi-processor compilation” to “Yes”
Get the most out of your machine!
“Configuration Properties” ➜ “C/C++” ➜ “Optimization”
★ Set “Whole Program Optimization” to “Yes”
Get the most out of your code!
“Configuration Properties” ➜ “C/C++” ➜ “Preprocessor”
★ Set “Use Standard Conforming Preprocessor” to “Yes”
Of course, we want to use a standard conforming preprocessor and not a Microsoft specific dialect.
“Configuration Properties” ➜ “C/C++” ➜ “Code Generation”
★ Set “Enable String pooling” to “Yes”
If you often use the same string literal more than once in your code, this will optimize it for you that the same string literal only occurs once and use the same address. This is very helpful, e.g., when you often use “Yes” and “No” or “true” and “false” and so on.
★ Set “Enable C++ Exceptions” to “Yes”
C++ without exceptions is not C++.
“Configuration Properties” ➜ “C/C++” ➜ “Language”
★ Set “Disable Language extension” to “No”
This might look like a controversial decision by me. Don’t we want to be standard conform?
Of course, we want! But this setting is very, VERY special. It is for the C compiler and not the C++ compiler. If you compile real C files with .c as extension, then a setting to “Yes” will run the C compiler in strict C89(!) mode. But when compile C++ files a completely different mode is used in the compiler: C++11 and newer requires C99 feature level. For this reason, for C++ code, it is absolutely NOT recommended to set this setting to “Yes”. It may lead to unexpected behavior otherwise! (See Microsoft documentation: https://learn.microsoft.com/en-us/cpp/build/reference/za-ze-disable-language-extensions )
★ Set “Treat wchar_t as built-in type” to “Yes” (is normally the default now.)
wchar_t
should be a distinct type rather than an unsigned short
.
★ Set “Force Conformance in for loop scope” to “Yes” (is the default now.)
The for loop opening brackets will start a new scope for the declared variables as it is specified by the C++ standard. If you need the variable after the for loop, you must declare it outside the loop.
★ Set “Enforce Type conversion rules” to “Yes”
This will disable VS specific cast rules which are not C++11 conformant.
★ Set “Enable Run-Time Type information” to “Yes”
Of course, we want to have RTTI, otherwise dynamic_cast and typeid are not available.
★ Set “C++ Language standard” to “ISO C++20”
Use the latest and greatest C++ standard version. Note: Be aware that you need at least Visual Studio 2022 17.2 for have the C++20 standard including all fixed Standard Defect Reports. (For Visual Studio 2019 you need at least 16.11.14.)
I recommend to use “C++ Latest” only for experiments and not for real projects.
★ Set “C Language standard” to “ISO C17”
Use the latest and greatest C standard.
★ Set “Conformance Mode” to “Yes”
This is important for disable various VS specific language dialects. This will especially disable passing anonymous objects as non-const reference parameters to functions, which is not standard conform and will produce a lot of compile errors on other compilers and platforms.
struct Object {}
void test( Object & rObj ) // non const reference
{
}
// invoke function with anonymous/temporary object.
test( Object{} ); // compiles without conformance mode but should not!
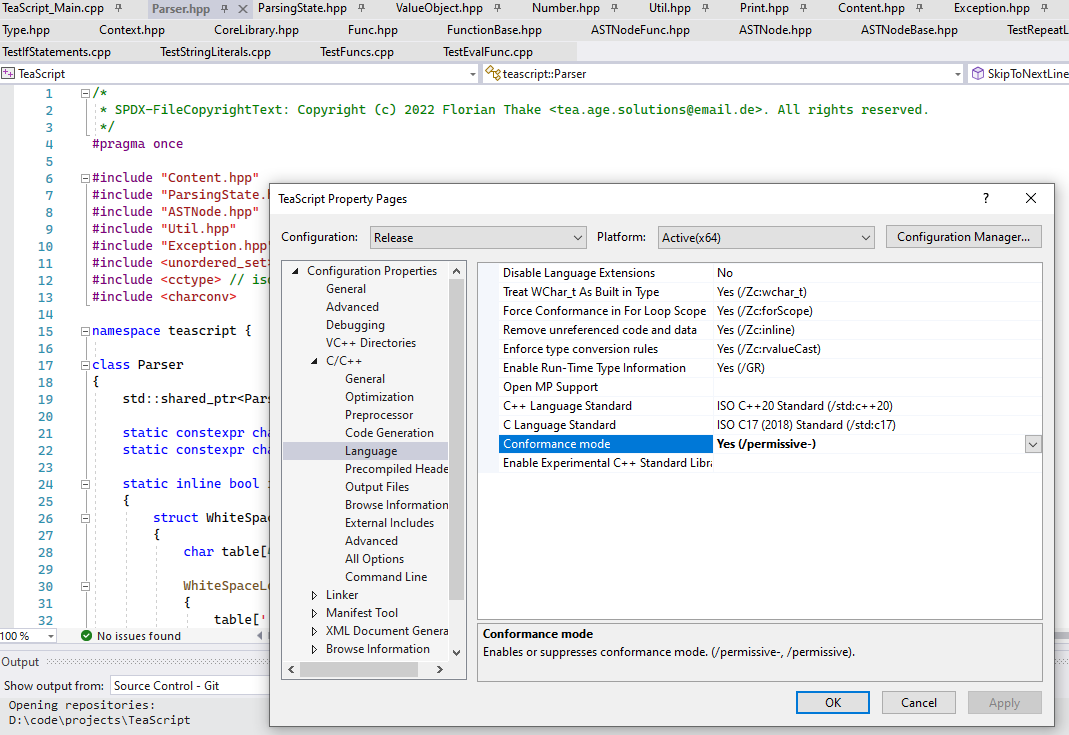
“Configuration Properties” ➜ “C/C++” ➜ “Command Line”
Add the following to the command line:
★ /Zc:__cplusplus
This sets the correct value to the __cplusplus macro and you can use it directly for test it instead of use other VS specific macros.
★ /utf-8
Your source code is UTF-8. Then you can use Unicode characters in comments and string literals (while staying platform independent!).
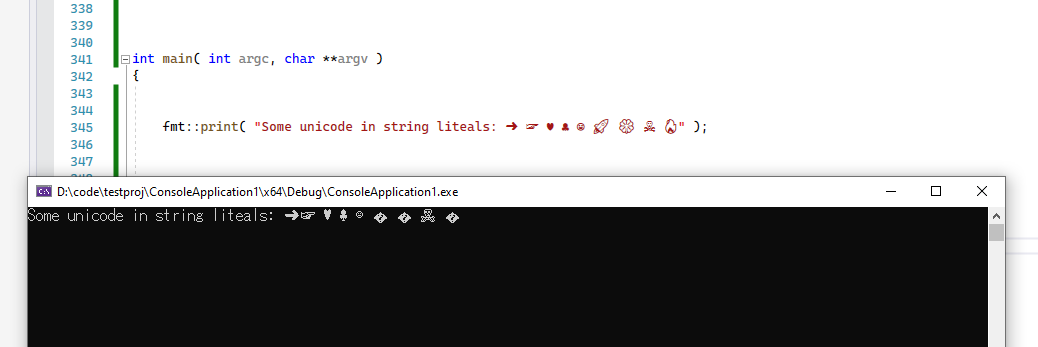
That was it. Did I miss one important? Feel free to leave a comment, also when you like it or disagree with some setting. Happy coding! 😊